C-Programming:-
In this tutorial you will learn:-
Bitwise operator :-
In C programming the following 6 operator are bitwise operator are:-
1. &( Bitwise AND ):-
(bitwise AND ) takes two number and does AND on every bit of two number . The result of AND is 1 only of both bits are 1 .
2. | ( Bitwise OR ) :-
The | (bitwise OR ) in C takes two numbers as operands and does OR every bit of two numbers the result of OR is 1 if any of the two bits is 1 .
3. ^ ( Bitwise XOR ):-
( Bitwise XOR ) takes two number and does XOR on every bit of two numbers .
4. << ( Left shift ) :-
The << ( Left shift ) in C takes two numbers , left shifts the bits of the first operands , the second operands decides the numbers of places to shift.
5. >> ( Right shift ) :-
( Right shift ) takes two numbers , right shift the bits of the first number the seconds number decides the number of place to shift .
6. ~ ( Bitwise NOT ):-
The ~ ( Bitwise NOT ) in C takes one number and inverts all bits of it.
Note point:-
* The left shift and right shift operators should not be used for negative number . if any of the operands is a negative number it result in undefined behaviour .
for example :- -1 << 1 and 1 << -1 is undefined.
* The bitwise XOR operator is the most useful operator from technical interview perspective
It is used in many problems .
A simple example is:-
A set of number where all element occur even number of time except one number find odd occuring number this problem can be efficiently solved by just doing XOR of all numbers.
#include<stdio.h>
int findodd( int arr [] , int n )
{ // user define method
int res = 0 , i ;
for ( i=0 ; i<n ; i++)
{
res ^ = arr[i];
return(0);
}
// Driver mode :-
#include<stdio.h>
int main ( void )
{
int arr [] = { 12 , 12 , 14 , 90 , 14 , 14 , 14 };
int n = sizeof(arr) , sizeof(arr[0]);
printf("The odd occuring element is = %d",find odd (arr,n));
return (0);
}
OUTPUT:-
The odd occuring element is = 90
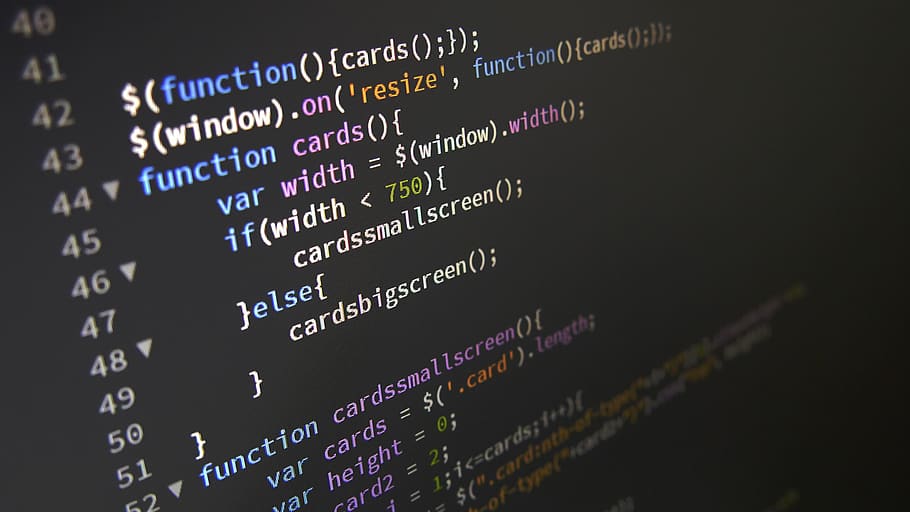
0 comments:
Post a Comment
If you have any doubt, please let me know